MY BLOG FOR NEXT JS
2.77 min read | May 02, 2023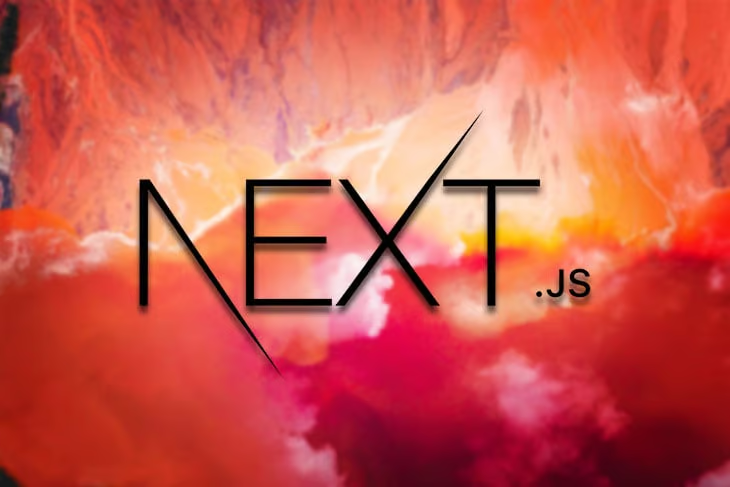
My Blog for Next.js
Welcome to Abhaya's Blog where you get access to all the latest tech Abhaya is learning and sharing. This is the starter course for people learning and starting out to Next.js
Next.js is a popular framework for building server-side rendered React applications. With Next.js, you can build high-performance, SEO-friendly web applications with ease. In this tutorial, we'll walk you through the process of creating a blog using Next.js.
🖊️Getting Started with Next.js
To get started with Next.js, you first need to create a new Next.js project. You can do this using the create-next-app
command:
npx create-next-app my-blog
Once you've created your Next.js project, you can start building your blog.
➕Creating Pages in Next.js
In Next.js, you can create pages using the pages
directory. Each file in the pages
directory represents a page in your application. For example, you can create a pages/index.js
file to represent the home page of your blog.
function HomePage() {
return <h1>Welcome to my blog!</h1>;
}
export default HomePage;
In the above example, we've created a simple home page component that displays a welcome message.
Using Dynamic Routing in Next.js
Next.js allows you to use dynamic routing to create dynamic pages in your application. You can do this by creating a file with square brackets in the pages
directory. For example, you can create a pages/posts/[slug].js
file to represent a dynamic post page.
import { useRouter } from "next/router";
function PostPage() {
const router = useRouter();
const { slug } = router.query;
return <h1>Post: {slug}</h1>;
}
export default PostPage;
In the above example, we've created a dynamic post page component that uses the useRouter
hook to get the current post slug from the router query.
Fetching Data in Next.js
Next.js allows you to fetch data for your pages using the getStaticProps
and getServerSideProps
functions. getStaticProps
is used to fetch data at build time, while getServerSideProps
is used to fetch data at runtime.
function HomePage({ posts }) {
return (
<>
<h1>Welcome to my blog!</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</>
);
}
export async function getStaticProps() {
const res = await fetch("https://jsonplaceholder.typicode.com/posts");
const posts = await res.json();
return {
props: {
posts,
},
};
}
In the above example, we've used getStaticProps
to fetch a list of posts from a JSON API and pass them as a prop to the home page component.
Conclusion
Next.js is a powerful framework for building server-side rendered React applications. In this tutorial, we've walked you through the process of creating a blog using Next.js. With its powerful features and flexible API, Next.js is a valuable tool for building modern and performant web applications.
P.s. :- This Blog is not written by me but a dummy blog generated by ChatGPT